How to Create a Chat App in Android: A Comprehensive Guide
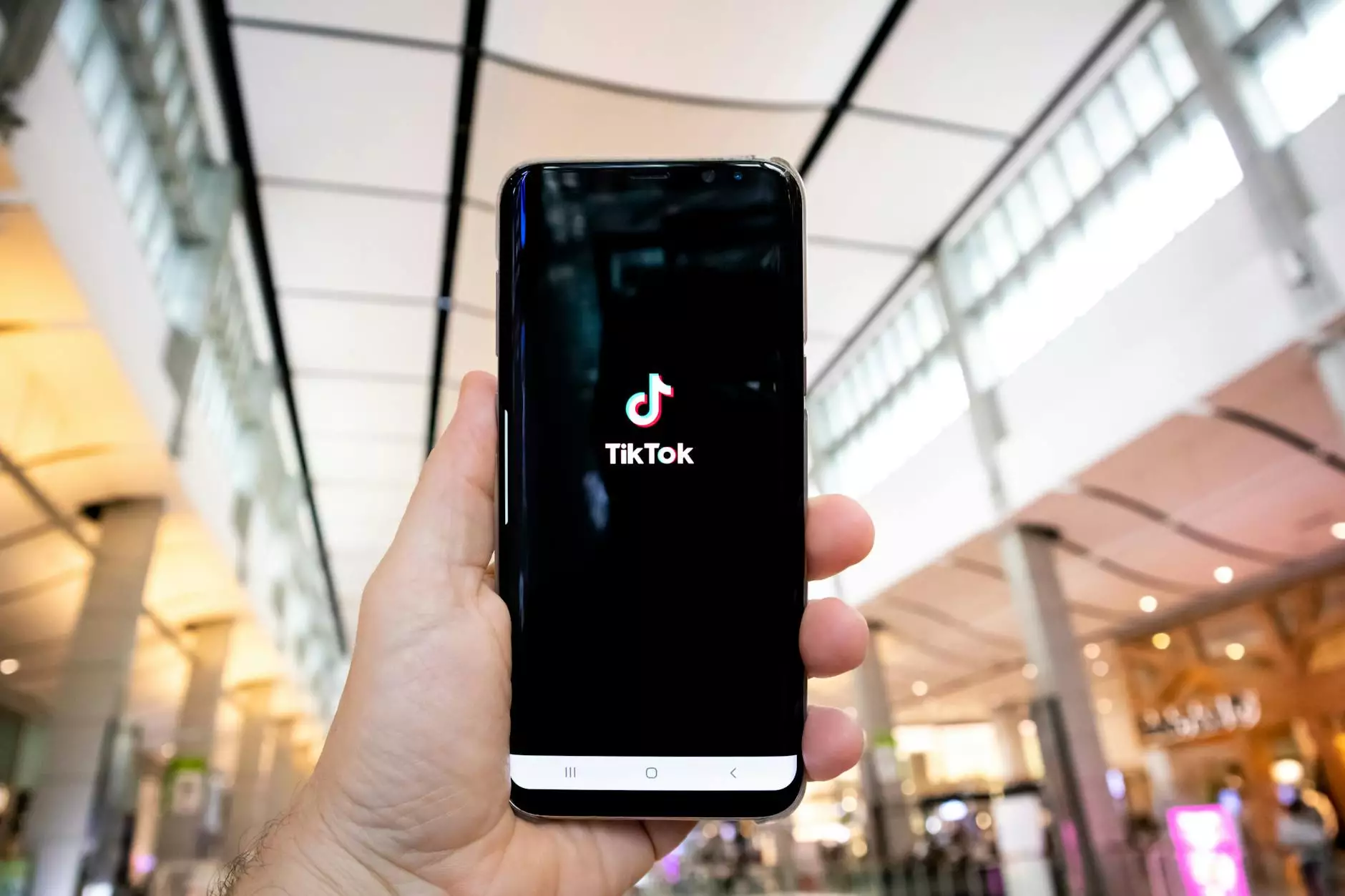
In today’s digital age, chatting applications have become an integral part of our daily communication. Whether it's for personal use or business, the demand for sophisticated chat applications has skyrocketed. It’s no surprise that many aspiring developers are eager to learn how to create a chat app in Android. This guide will walk you through the entire process, helping you unleash your creativity and technical skills.
Understanding the Basics of a Chat Application
Before diving into the technical aspects, it’s crucial to understand the fundamental components that make up a chat application. A typical chat app generally consists of the following:
- User Registration and Authentication: Users should be able to create accounts and log in securely.
- Real-time Messaging: Users must send and receive messages in real-time.
- Chat Rooms or One-on-One Chats: Users can communicate in groups or individually.
- Notification System: Users should receive notifications for new messages.
- User Profiles: Each user should have a profile that can be customized.
Choosing the Right Technology Stack
When considering how to create a chat app in Android, choosing the right technology stack is essential. Here are some of the primary technologies you might consider:
- Programming Language: Java or Kotlin are the most recommended languages for Android development.
- Frameworks: Use Android Jetpack components to simplify your development process.
- Real-Time Communication: Firebase Cloud Messaging (FCM) or WebSocket can provide real-time messaging capabilities.
- Database: Firebase Firestore or SQLite can be considered for storing user data and messages.
Setting Up Your Development Environment
To get started, you need a proper development environment. Follow these steps to set up your Android studio:
- Download and install Android Studio.
- Open Android Studio and start a new project.
- Select a template for your app; the "Empty Activity" is a good starting point.
- Configure your project settings (Name, Package name, Save location, Language).
- Click 'Finish' and wait for Android Studio to set up your project.
Implementing User Registration and Authentication
The first major functionality to implement in your chat app is user registration and authentication. You can use Firebase Authentication for this purpose:
- Integrate Firebase into your project by adding the Firebase SDK.
- Enable Firebase Authentication from the Firebase console.
- Create functions for user registration and login using Firebase's API.
Here’s a sample code snippet for user registration:
FirebaseAuth auth = FirebaseAuth.getInstance(); auth.createUserWithEmailAndPassword(email, password) .addOnCompleteListener(this, new OnCompleteListener() { @Override public void onComplete(@NonNull Task task) { if (task.isSuccessful()) { // Registration successful } else { // Registration failed } } });Designing the User Interface
A visually appealing user interface is key to keeping users engaged. Consider using the following UI elements:
- RecyclerView: For displaying messages in a chat layout.
- EditText: For message input.
- Button: For sending messages.
- ImageView: To display user profile pictures.
Utilize XML layout files to structure your UI. Here’s an example of a simple chat interface layout:
Real-Time Messaging Implementation
The heart of any chat application lies in its messaging feature. To implement real-time messaging, you can utilize Firebase Realtime Database or Firestore:
- Set up your chosen database in Firebase.
- Create a data structure to handle chat messages (e.g., sender ID, message content, timestamp).
- Add listeners to your database to fetch and display messages in real-time.
Sample Code for Sending Messages:
DatabaseReference databaseReference = FirebaseDatabase.getInstance().getReference("messages"); String messageId = databaseReference.push().getKey(); Message message = new Message(senderId, messageContent, timestamp); databaseReference.child(messageId).setValue(message);Implementing Notifications
To keep users engaged, implement a notification system that alerts them of new messages. You can utilize Firebase Cloud Messaging (FCM) for this purpose:
- Set up FCM in your Firebase project.
- Implement a service to handle incoming messages.
- Create a notification when a new message is received.
Example Code for Sending Notifications:
FirebaseMessaging.getInstance().send(new RemoteMessage.Builder(targetToken) .setNotification(new Notification("New Message", messageContent)) .build());User Profiles Management
Providing users with customizable profiles enhances their experience. Here’s how to implement it:
- Allow users to upload profile pictures and set display names.
- Store user profile data in the database.
- Fetch and display user profiles in chats.
Sample Code for Updating User Profile:
DatabaseReference userRef = FirebaseDatabase.getInstance().getReference("users").child(userId); userRef.child("displayName").setValue(displayName); userRef.child("profilePicture").setValue(profilePictureUrl);Testing Your Chat App
Once your app is complete, testing is crucial. Consider the following:
- Unit Testing: Test individual components of your app.
- User Acceptance Testing: Ensure real users find the app functional and enjoyable.
- Performance Testing: Evaluate your app’s performance under various conditions.
Deployment and Future Enhancements
After successful testing, it’s time to deploy your app on the Google Play Store. Here’s a quick guide:
- Create a developer account on the Google Play Store.
- Prepare promotional material (screenshots, descriptions).
- Upload your APK file and fill in the required information.
- Publish your app and promote it through various channels.
Conclusion
Building a chat application for Android is a rewarding journey that combines your coding skills with creativity. This guide has laid the groundwork on how to create a chat app in Android, from conceptualization and design to implementation and deployment. Remember to prioritize user experience and continuously seek feedback for improvement. As you develop your app, consider future enhancements like adding voice and video calling, rich media sharing, or even integrating AI features to stay ahead in the competitive market.
With dedication and the right approach, you can create a successful chat application that meets user needs and stands out in the crowded app marketplace. Visit nandbox.com for more insights on mobile application development!